Q1. Types of Popup in Selenium ?
Ans: There are the four methods that we would be using along with the Alert interface.
Q5 What are the four Pillars of Java ? Explain ?
Ans:
Ans: There are the four methods that we would be using along with the Alert interface.
1) void dismiss() – The dismiss() method clicks on the “Cancel” button as soon as the pop up window appears.
2) void accept() – The accept() method clicks on the “Ok” button as soon as the pop up window appears.
3) String getText() – The getText() method returns the text displayed on the alert box.
4) void sendKeys(String stringToSend) – The sendKeys() method enters the specified string pattern into the alert box.
2) void accept() – The accept() method clicks on the “Ok” button as soon as the pop up window appears.
3) String getText() – The getText() method returns the text displayed on the alert box.
4) void sendKeys(String stringToSend) – The sendKeys() method enters the specified string pattern into the alert box.
Alert alert = driver.switchTo().alert();
alert.accept();
System.out.println(driver.switchTo().alert().getText());
driver.switchTo().alert().accept();
Q2. Which kind of Application you are working on ?
Ans: Web- Based Application.
Types of Applications:
- Mobile Applications
- Desktop GUI Applications
- Web-based Applications
- Enterprise Applications
- Scientific Applications
- Gaming Applications
- Big Data technologies
- Business Applications
- Distributed Applications
- Cloud-based Applications
Q3 . Difference Between Junit and TestNG FW ?
JUnit | TestNG |
It is opensource testing framework. | It is also opensource testing framework with added benefits. |
Does not support advanced annotations. | Supports advanced annotations and special annotations. |
Does not support parallel testing. | Supports Parallel testing. |
Group test feature is not present here. | Group test feature is TestNG's commonly used feature. |
Parameterized test case take less time. | Parameterized test take more time and are complicated. |
It is difficult to use. | It is much easier to use. |
Q4. With Cucumber can we use TestNG
Ans: Yes, definitely we can use TestNG along with Cucumber. We have already used the same in different frameworks while working for automation testing company. Below is the sample code of Cucumber runner file with TestNG and you can call it from TestNG suite,
Runner file:
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import cucumber.api.CucumberOptions;
import cucumber.api.testng.AbstractTestNGCucumberTests;
import driver.Driver;
@CucumberOptions(
strict = true,
features = "src/test/java/features",
glue = "stepDefinations",
plugin = {"pretty", "html:target/cucumber-html-report" },
tags={"@login1"}
)
public class CucumberRunWithTestNG extends AbstractTestNGCucumberTests{
@BeforeClass
public void instantiateDriver(){
Driver.getDriver();
}
@AfterClass
public void tearDown(){
Driver.getDriver().quit();
}
}
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import cucumber.api.CucumberOptions;
import cucumber.api.testng.AbstractTestNGCucumberTests;
import driver.Driver;
@CucumberOptions(
strict = true,
features = "src/test/java/features",
glue = "stepDefinations",
plugin = {"pretty", "html:target/cucumber-html-report" },
tags={"@login1"}
)
public class CucumberRunWithTestNG extends AbstractTestNGCucumberTests{
@BeforeClass
public void instantiateDriver(){
Driver.getDriver();
}
@AfterClass
public void tearDown(){
Driver.getDriver().quit();
}
}
TestNG suite file:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Testng Cucumber Suite" parallel="none">
<test name="Run All Tests">
<classes>
<class name="runners.CucumberRunWithTestNG"></class>
<!-- <class name="main.AnotherRunner"></class> This class could be used to run multiple runner classes-->
</classes>
</test> <!-- Test -->
</suite> <!-- Suite -->
<suite name="Testng Cucumber Suite" parallel="none">
<test name="Run All Tests">
<classes>
<class name="runners.CucumberRunWithTestNG"></class>
<!-- <class name="main.AnotherRunner"></class> This class could be used to run multiple runner classes-->
</classes>
</test> <!-- Test -->
</suite> <!-- Suite -->
Q5 What are the four Pillars of Java ? Explain ?
Ans:
- Abstraction is the process of exposing the essential details of an entity, while ignoring the irrelevant details, to reduce the complexity for the users.
- Encapsulation is the process of bundling data and operations on the data together in an entity.
- Inheritance is used to derive a new type from an existing type, thereby establishing a parent-child relationship.
- Polymorphism lets an entity take on different meanings in different contexts.
Q 6. Is it necessary to use super keyword for calling parent class Constructor ?
Ans: Yes,
A subclass can have its own private data members, so a subclass can also have its own constructors.
The constructors of the subclass can initialize only the instance variables of the subclass. Thus, when a subclass object is instantiated the subclass object must also automatically execute one of the constructors of the superclass.
To call a superclass constructor the super keyword is used.
Q 6. Can we Automate OTP via email ?
Ans: Yes !
Once you send OTP from the application. You have to use
javax.mail
and have to write the code to read the email from mailbox for provided emailid. and then extract the OTP using suitable regex from content you get.
First add these dependency if you are using Maven project otherwise you have to add same libraries in your project
<!-- https://mvnrepository.com/artifact/javax.mail/mail -->
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
Create one class and use this code :
String hostName = "smtp.gmail.com";
String username = "email username"
String password = "email passeord"
int messageCount;
int unreadMsgCount;
String emailSubject;
Message emailMessage;
public MailReader() {
Properties sysProps = System.getProperties();
sysProps.setProperty("mail.store.protocol", "imaps");
try {
Session session = Session.getInstance(sysProps, null);
Store store = session.getStore();
store.connect(hostName, username, password);
Folder emailInbox = store.getFolder("INBOX");
emailInbox.open(Folder.READ_WRITE);
messageCount = emailInbox.getMessageCount();
System.out.println("Total Message Count: " + messageCount);
unreadMsgCount = emailInbox.getNewMessageCount();
System.out.println("Unread Emails count:" + unreadMsgCount);
emailMessage = emailInbox.getMessage(messageCount);
emailSubject = emailMessage.getSubject();
Pattern linkPattern = Pattern.compile("href=\"(.*)\" target"); // here you need to define regex as per you need
Matcher pageMatcher =
linkPattern.matcher(emailMessage.getContent().toString());
while (pageMatcher.find()) {
System.out.println("Found OTP " + pageMatcher.group(1));
}
emailMessage.setFlag(Flags.Flag.SEEN, true);
emailInbox.close(true);
store.close();
} catch (Exception mex) {
mex.printStackTrace();
}
}
Q7. You are working on eCommerce application how you login ? Is there no Security ?
Ans:
- Use SSL and Ensure That Your Ecommerce web site is PCI Compliant
- An SSL is a digital certificate that encrypts information sent between a web server and web browser. It is one of the most effective ways to achieve data security on your eCommerce website and to keep your customer data protected. SSL also prompts customers that your website is secure enough to grant their credit card data.
PCI compliance is a security requirement created by major credit card brands in an attempt to reduce fraud and increase Ecommerce website security. The Payment Card Industry Data Security Standard (PCI DSS) applies to all companies who process, transmit and store payment card data online.
SSL. Stands for "Secure Sockets Layer." SSL is a secure protocol developed for sending information securely over the Internet. Many websites use SSL for secure areas of their sites, such as user account pages and online checkout. Usually, when you are asked to "log in" on a website, the resulting page is secured by SSL
The Payment Card Industry Data Security Standard is an information security standard for organizations that handle branded credit cards from the major card schemes. The PCI Standard is mandated by the card brands but administered by the Payment Card Industry Security Standards Council.
Q8 What is Bundle ID ?
Ans : A Bundle ID is a tool Apple uses to identify individual apps. ... You can change your Bundle ID on iTunes Connect until your app has been approved by Apple and is available on the App Store. Once your app is approved and available for download on the Apple App Store, your Bundle ID cannot be changed.
Q9. Which is the fastest method getText() or getAttribute() ?
Ans:
Q10. Suppose I hit a URL say www.facebook.com then I am on Homepage of it, As we know we are having many data on that page photos, titles, etc. So can we fetch all this data without hitting a Url ? How ?
Note: I am using that url but not hitting that url. can get all the data.
Ans: Yes, you can do that using headless.
1. HtmlUnitDriver – the initial headless driver
2. PhantomJS
PhantomJS is one of the best options. A sample example taken from here.
Q11. Limitation of Selenium ?
Ans:
Q10. Suppose I hit a URL say www.facebook.com then I am on Homepage of it, As we know we are having many data on that page photos, titles, etc. So can we fetch all this data without hitting a Url ? How ?
Note: I am using that url but not hitting that url. can get all the data.
Ans: Yes, you can do that using headless.
1. HtmlUnitDriver – the initial headless driver
2. PhantomJS
PhantomJS is one of the best options. A sample example taken from here.
var page = require('webpage').create(),
url = 'http://lite.yelp.com/search?find_desc=pizza&find_loc=94040&find_submit=Search';
page.open(url, function (status) {
if (status !== 'success') {
console.log('Unable to access network');
} else {
var results = page.evaluate(function() {
var list = document.querySelectorAll('address'), pizza = [], i;
for (i = 0; i < list.length; i++) {
pizza.push(list[i].innerText);
}
return pizza;
});
console.log(results.join('\n'));
}
phantom.exit();
});
OR
Don't use Selenium for this.
Use Jsoup.
100% headless, and does not require any 3rd party programs, unlike selenium, to get the page content.
Q11. Limitation of Selenium ?
Ans:
- Selenium does not support automation testing for desktop applications.
- Selenium requires high skill sets in order to automate tests more effectively.
- Since Selenium is open source software, you have to rely on community forums to get your technical issues resolved.
- We can't perform automation tests on web services like SOAP or REST using Selenium.
- We should know at least one of the supported programming languages to create tests scripts in Selenium WebDriver.
- It does not have built-in Object Repository like UTF/QTP to maintain objects/elements in centralized location. However, we can overcome this limitation using Page Object Model.
- Selenium does not have any inbuilt reporting capability; you have to rely on plug-ins like JUnit and TestNG for test reports.
- It is not possible to perform testing on images. We need to integrate Selenium with Sikuli for image based testing.
- Creating test environment in Selenium takes more time as compared to vendor tools like UFT, RFT, Silk test, etc.
- No one is responsible for new features usage; they may or may not work properly.
- Selenium does not provide any test tool integration for Test Management.
- Using Selenium tool alone mobile applications cannot be tested, with use of Appium we can achieve it.
- The Windows-based popup cannot be tested using Selenium alone we need to use AutoIT to handle the windows based popups.
Q12. Difference between annotations of Junit and TestNG ?
Ans:
Feature
|
JUnit 5
|
TestNG
|
Test annotation
|
@Test
|
@Test
|
Execute before the first test method in the current class is invoked
|
@BeforeAll
|
@BeforeClass
|
Execute after all the test methods in the current class have been run
|
@AfterAll
|
@AfterClass
|
Execute before each test method
|
@BeforeEach
|
@BeforeMethod
|
Execute after each test method
|
@AfterEach
|
@AfterMethod
|
Ignore Test
|
@ignore
|
@Test(enable=false)
|
Expected exception
|
@Test(expected=Arithmetic
Exception.class) |
@Test(expectedException=Arithmetic
Exception.class) |
Timeout
|
@Test(timeout = 1000)
|
@Test(timeout = 1000)
|
Execute before all tests in this suite have run
|
N/A
|
@BeforeSuite
|
Execute after all tests in this suite have run
|
N/A
|
@AfterSuite
|
Execute before the test
|
N/A
|
@BeforeTest
|
Execute after the test
|
N/A
|
@AfterTest
|
Execute before first test method that belongs to any of these groups is invoked
|
N/A
|
@BeforeGroups
|
Execute after the last test method that belongs to any of these groups is invoked
|
N/A
|
@AfterGroups
|
Q13. Difference between @test and @BeforeClass in TEstNG ?
Ans: @BeforeClass: The annotated method will be run before the first test method in the current class is invoked. @BeforeTest: The annotated method will be run before any test method belonging to the classes inside the <test> tag is run. Both the above TestNG annotations look similar in functionality.
Q14. What is Object repository ?
Ans: Object Repository is used to store element locator values in a centralized location instead of hard coding them within the scripts. We do create a property file (. properties) to store all the element locators and these property files act as an object repository in Selenium WebDriver.
Q15. Explain Exception Handling ? How can we achieve ?
Ans:
What is an exception?
An Exception is an unwanted event that interrupts the normal flow of the program. When an exception occurs program execution gets terminated. In such cases we get a system generated error message. The good thing about exceptions is that they can be handled in Java. By handling the exceptions we can provide a meaningful message to the user about the issue rather than a system generated message, which may not be understandable to a user.
Why an exception occurs?
There can be several reasons that can cause a program to throw exception. For example: Opening a non-existing file in your program, Network connection problem, bad input data provided by user etc.
Exception Handling
If an exception occurs, which has not been handled by programmer then program execution gets terminated and a system generated error message is shown to the user. For example look at the system generated exception below:
An exception generated by the system is given below
An exception generated by the system is given below
Exception in thread "main" java.lang.ArithmeticException: / by zero at ExceptionDemo.main(ExceptionDemo.java:5)
ExceptionDemo : The class name
main : The method name
ExceptionDemo.java : The filename
java:5 : Line number
This message is not user friendly so a user will not be able to understand what went wrong. In order to let them know the reason in simple language, we handle exceptions. We handle such conditions and then prints a user friendly warning message to user, which lets them correct the error as most of the time exception occurs due to bad data provided by user.
Advantage of exception handling
Exception handling ensures that the flow of the program doesn’t break when an exception occurs. For example, if a program has bunch of statements and an exception occurs mid way after executing certain statements then the statements after the exception will not execute and the program will terminate abruptly.
By handling we make sure that all the statements execute and the flow of program doesn’t break.
By handling we make sure that all the statements execute and the flow of program doesn’t break.
Difference between error and exception
Errors indicate that something severe enough has gone wrong, the application should crash rather than try to handle the error.
Exceptions are events that occurs in the code. A programmer can handle such conditions and take necessary corrective actions. Few examples:
NullPointerException – When you try to use a reference that points to null.
ArithmeticException – When bad data is provided by user, for example, when you try to divide a number by zero this exception occurs because dividing a number by zero is undefined.
ArrayIndexOutOfBoundsException – When you try to access the elements of an array out of its bounds, for example array size is 5 (which means it has five elements) and you are trying to access the 10th element.
NullPointerException – When you try to use a reference that points to null.
ArithmeticException – When bad data is provided by user, for example, when you try to divide a number by zero this exception occurs because dividing a number by zero is undefined.
ArrayIndexOutOfBoundsException – When you try to access the elements of an array out of its bounds, for example array size is 5 (which means it has five elements) and you are trying to access the 10th element.
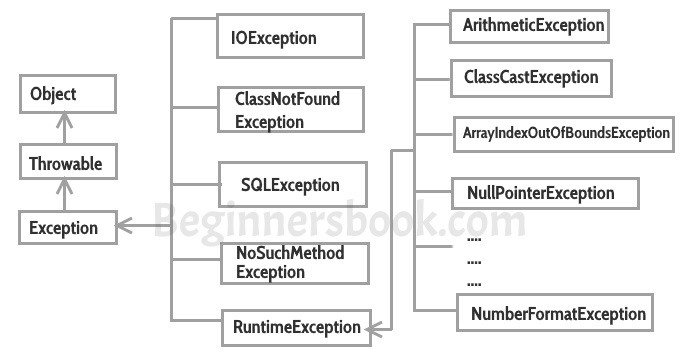
Types of exceptions
There are two types of exceptions in Java:
1)Checked exceptions
2)Unchecked exceptions
1)Checked exceptions
2)Unchecked exceptions
Q16. What are Different kind of Exceptions you have faced ?
Ans: The complete list of exceptions in Selenium
1. ConnectionClosedException: This exception takes place when there is a disconnection in the driver.
2. ElementClickInterceptedException: The command could not be completed as the element receiving the events is concealing the element which was requested clicked.
3. ElementNotInteractableException: This Selenium exception is thrown when an element is presented in the DOM but it is impossible to interact with such element.
4. ElementNotSelectableException: This Selenium exception is thrown when an element is presented in the DOM but you can be able to select. Hence, it is impossible to interact with.
5. ElementNotVisibleException: This type of Selenium exception takes place when existing element in DOM has a feature set as hidden. In this situation, elements are there, but you can not see and interact with the WebDriver.
6. ErrorHandler.UnknownServerException: Exception is used as a placeholder if the server returns an error without a stack trace.
7. ErrorInResponseException: This exception is thrown when a fault has occurred on the server side. You can see it happens when interacting with the Firefox extension or the remote driver server.
8. ImeActivationFailedException: This exception occurs when IME engine activation has failed.
9. ImeNotAvailableException: This exception takes place when IME support is unavailable.
10. InsecureCertificateException: Navigation made the user agent to hit a certificate warning, which is caused by an invalid or expired TLS certificate.
11. InvalidArgumentException: This Selenium exception is thrown if an argument does not belong to the expected type.
12. InvalidCookieDomainException: This happens when you try to add a cookie under a different domain rather than the current URL.
13. InvalidCoordinatesException: This happens if the coordinates offered to an interacting operation are not valid.
14. InvalidElementStateException: This Selenium exception occurs if a command cannot be finished as the element is invalid.
15. InvalidSessionIdException: Takes place when the given session ID is not included in the list of active sessions, which means the session does not exist or is inactive either.
16. InvalidSwitchToTargetException: Happens if frame or window target to be switched does not exist.
17. JavascriptException: This problem happens when executing JavaScript supplied by the user.
18. JsonException: Happens when you afford to get the session capabilities where the session is not created.
19. MoveTargetOutOfBoundsException: Takes place if the target provided to the ActionChains move() methodology is not valid. For example: out of document.
20. NoAlertPresentException: Happens when you switch to no presented alert.
21. NoSuchAttributeException: Occurs when the attribute of element could not be found.
22. NoSuchContextException: Happens in mobile device testing and is thrown by ContextAware.
23. NoSuchCookieException: This exception is thrown if there is no cookie matching with the given path name found amongst the associated cookies of the current browsing context’s active document.
24. NoSuchElementException: Happens if an element could not be found.
25. NoSuchFrameException: Takes place if frame target to be switch does not exist.
26. NoSuchWindowException: Occurs if window target to be switch does not exist.
27. NotFoundException: This exception is subclass of WebDriverException. It happens when an element on the DOM does not exist.
28. RemoteDriverServerException: This Selenium exception is thrown when server do not respond due to the problem that the capabilities described are not proper.
29. ScreenshotException: It is impossible to capture a screen.
30. ScriptTimeoutException: Thrown when executeAsyncScript takes more time than the given time limit to return the value.
31. SessionNotCreatedException: A new session could not be successfully created.
32. SessionNotFoundException: The WebDriver is performing the action right after you quit the browser.
33. StaleElementReferenceException: This Selenium exception happens if the web element is detached from the current DOM.
34. TimeoutException: Thrown when there is not enough time for a command to be completed.
35. UnableToCreateProfileException: You can open a browser with certain options using profiles, but sometimes a new version of Selenium driverserver or browser may not support the profiles.
36. UnableToSetCookieException: Occurs if a driver is unable to set a cookie.
37. UnexpectedAlertPresentException: This Selenium exception happens when there is the appearance of an unexpected alert.
38. UnexpectedTagNameException: Happens if a support class did not get a web element as expected.
39. UnhandledAlertException: It happens when there is an alert, but WebDriver is unable to perform Alert operation.
40. UnknownMethodException: Thrown when the requested command matching with a known URL but not matching with a methodology for that URL.
41. UnreachableBrowserException: This Selenium exception happens if the browser is unable to be opened or have crashed because of some reasons.
42. UnsupportedCommandException: Occurs when remote WebDriver does not send valid command as expected.
43. WebDriverException: This takes place when the WebDriver is performing the action right after you close the browser.
Q17. Explain Null pointer Exception ?
Ans: Java NullPointerException is an unchecked exception and extends
1. Why NullPointerException occur in the code
NullPointerException is a situation in code where you try to access/ modify an object which has not been initialized yet. It essentially means that object reference variable is not pointing anywhere and refers to nothing or ‘null‘. A example java program which throws null pointer exception.
package com.howtodoinjava.demo.npe;
public class SampleNPE
{
public static void main(String[] args)
{
String s = null;
System.out.println( s.toString() ); // 's' is un-initialized and is null
}
}
2. Common places where Java NullPointerException usually occur
Well, NullPointerException can occur anywhere in the code for various reasons but I have prepared list of most frequent places based on my experience.
Invoking methods on an object which is not initialized
Parameters passed in a method are null
Calling toString() method on object which is null
Comparing object properties in if block without checking null equality
Incorrect configuration for frameworks like spring which works on dependency injection
Using synchronized on an object which is null
Chained statements i.e. multiple method calls in a single statement
This is not an exhaustive list. There are several other places and reasons also. If you can recall any such other, please leave a comment. it will help others (beginners) also.
3. Best ways to avoid Java NullPointerException
3.1. Ternary Operator
This operator results to the value on the left hand side if not null else right hand side is evaluated. It has syntax like :
boolean expression ? value1 : value2;
If expression is evaluated as true then entire expression returns value1 otherwise value2. Its more like if-else construct but it is more effective and expressive. To prevent NullPointerException (NPE) , use this operator like below code:
String str = (param == null) ? "NA" : param;
3.2. Use apache commons StringUtils for String operations
Apache commons lang is a collection of several utility classes for various king of operation. One of them is StringUtils.java. Use StringUtils.isNotEmpty() for verifying if string passed as parameter is null or empty string. If it is not null or empty; then use it further.
Other similar methods are StringUtils. IsEmpty(), and StringUtils.equals(). They claim in their javadocs that if StringUtils.isNotBlank() throws an NPE, then there is a bug in the API.
if (StringUtils.isNotEmpty(obj.getvalue())){
String s = obj.getvalue();
....
}
Q18. I have to add 3 different kinds of products to cart i.e. 3 different urls, 3 different xpath for add to cart button so how will you write the code ? How will you reduce the length of code ?
Ans: Java NullPointerException is an unchecked exception and extends
RuntimeException
. NullPointerException doesn’t force us to use catch block to handle it. This exception is very much like a nightmare for most of java developer community. They usually pop up when we least expect them.1. Why NullPointerException occur in the code
NullPointerException is a situation in code where you try to access/ modify an object which has not been initialized yet. It essentially means that object reference variable is not pointing anywhere and refers to nothing or ‘null‘. A example java program which throws null pointer exception.
package com.howtodoinjava.demo.npe;
public class SampleNPE
{
public static void main(String[] args)
{
String s = null;
System.out.println( s.toString() ); // 's' is un-initialized and is null
}
}
2. Common places where Java NullPointerException usually occur
Well, NullPointerException can occur anywhere in the code for various reasons but I have prepared list of most frequent places based on my experience.
Invoking methods on an object which is not initialized
Parameters passed in a method are null
Calling toString() method on object which is null
Comparing object properties in if block without checking null equality
Incorrect configuration for frameworks like spring which works on dependency injection
Using synchronized on an object which is null
Chained statements i.e. multiple method calls in a single statement
This is not an exhaustive list. There are several other places and reasons also. If you can recall any such other, please leave a comment. it will help others (beginners) also.
3. Best ways to avoid Java NullPointerException
3.1. Ternary Operator
This operator results to the value on the left hand side if not null else right hand side is evaluated. It has syntax like :
boolean expression ? value1 : value2;
If expression is evaluated as true then entire expression returns value1 otherwise value2. Its more like if-else construct but it is more effective and expressive. To prevent NullPointerException (NPE) , use this operator like below code:
String str = (param == null) ? "NA" : param;
3.2. Use apache commons StringUtils for String operations
Apache commons lang is a collection of several utility classes for various king of operation. One of them is StringUtils.java. Use StringUtils.isNotEmpty() for verifying if string passed as parameter is null or empty string. If it is not null or empty; then use it further.
Other similar methods are StringUtils. IsEmpty(), and StringUtils.equals(). They claim in their javadocs that if StringUtils.isNotBlank() throws an NPE, then there is a bug in the API.
if (StringUtils.isNotEmpty(obj.getvalue())){
String s = obj.getvalue();
....
}
Q18. I have to add 3 different kinds of products to cart i.e. 3 different urls, 3 different xpath for add to cart button so how will you write the code ? How will you reduce the length of code ?